SwiftUI ScrollView is a container view that allows users to scroll through a collection of views vertically, horizontally, or in both directions. It provides a way to display content that exceeds the available screen space, enabling users to view all the content by scrolling.
Key features of SwiftUI’s ScrollView include:
- Scroll Direction: ScrollView can be configured to scroll vertically, horizontally, or both, depending on the layout requirements of your app.
- Dynamic Content: It supports dynamic content, meaning you can add or remove views dynamically within the ScrollView, and it adjusts its scrolling behavior accordingly.
- Customization: ScrollView provides various customization options, such as content alignment, scroll indicators, and insets.
- Performance Optimization: SwiftUI’s ScrollView is designed to efficiently handle large amounts of content while maintaining good performance.
Vertical ScrollView
To create a vertical scroll view, use the following code snippet:
ScrollView(.vertical) {
VStack {
ForEach(1..<21) { index in
Text("Row \(index)")
}
.frame(width:200, height:100)
.background(.gray)
.foregroundColor(.white)
}
}
In this example:
- By default ScrollView has
.vertical
parameter for vertical scrolling. If we want to scroll only in vertical direction then we do not need to provide it as a parameter.
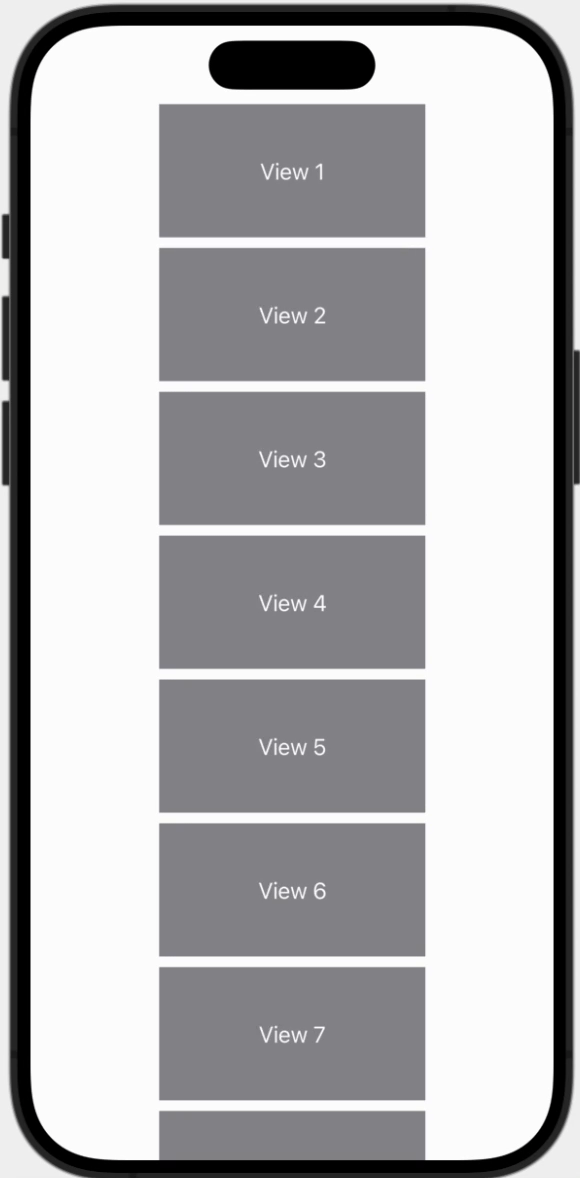
Horizontal ScrollView
To add a horizontal ScrollView in SwiftUI, you can use the .horizontal
parameter within the ScrollView initializer.
ScrollView(.horizontal) {
HStack {
ForEach(1..<21) { index in
Text("Row \(index)")
}
.frame(width:200, height:100)
.background(.gray)
.foregroundColor(.white)
}
}
By default scrollview adjusts its height according to the views added inside it. In this horizontal scrollview example its height will be 100. But you can change its height using frame modifier. Let us set the height equal to 400 and background colour to green so we can observe height of scrollview.
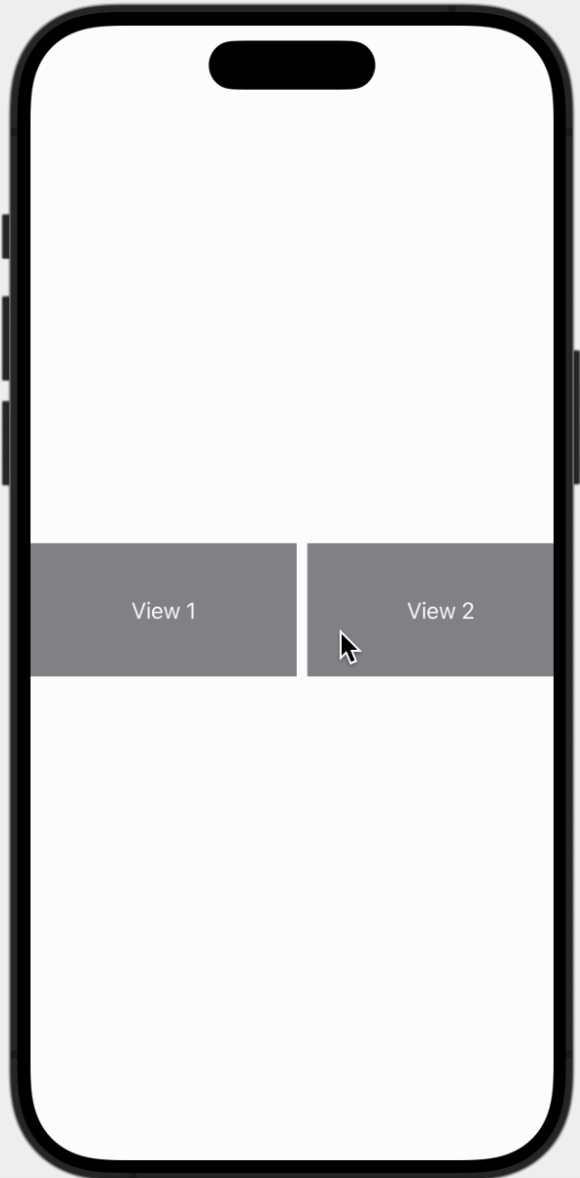
ScrollView(.horizontal) {
HStack {
ForEach(1..<21) { index in
Text("Row \(index)")
}
.frame(width:200, height:100)
.background(.gray)
.foregroundColor(.white)
}
}.frame(height:400).background(.green)
Scrolling Vertically and Horizontally
To enable scrolling on both horizontally and vertically, you can use a single ScrollView and allow it to scroll in both directions by setting its axes parameter to .horizontal
and .vertical
.
ScrollView([.horizontal, .vertical]) {
}
Hide ScrollView’s Indicators
If you want to hide the scroll indicators on scrolling, you can do it by simply providing parameter showIndicators: false
like
ScrollView(.horizontal, showsIndicators: false) {
}